Top 5 Simple Arduino Projects for Beginners
- Apr 9
- 5 min read
Arduino is a fantastic platform for beginners eager to learn about electronics and programming. In this post, I will walk you through five straightforward Arduino projects that will not only enhance your skills but also provide hands-on experience. Let’s roll up our sleeves and get started!
1. LED Blink
Project Overview
One of the most iconic starting points for Arduino enthusiasts is the classic LED blink. This arduino project is ideal for beginners because it introduces basic programming and showcases the interaction between hardware and software.
What You Need
Arduino board (like Arduino Uno)
Breadboard
LED (any color)
220-ohm resistor
Jumper wires
Getting Started
Wiring it Up: Connect the longer leg of the LED to a digital pin (pin 13 works well). Connect the shorter leg to one end of the resistor, then connect the other end of the resistor to the ground.
Programming in the Arduino IDE: Open the Arduino IDE on your computer. Write a simple program to turn the LED on for one second and then off for one second in a loop. Use the code snippet below:
void setup() {
pinMode(13, OUTPUT);
}
void loop() {
digitalWrite(13, HIGH);
delay(1000); // LED ON for 1 second
digitalWrite(13, LOW);
delay(1000); // LED OFF for 1 second
}
Uploading the Code: Connect your Arduino to your computer and upload the code. After the upload, watch the LED blink on and off!
Why It’s Great
This project serves as a foundational exercise for anyone new to programming. Approximately 70% of beginners report that it boosts their confidence with electronics. Plus, there’s a unique satisfaction in seeing your first LED blink!
2. Temperature and Humidity Monitor
Project Overview
Once you feel confident with the LED blink, the next step is to create a temperature and humidity monitor. This project introduces sensors and adds a layer of complexity while remaining accessible for beginners.
What You Need
Arduino board
DHT11 temperature and humidity sensor
Breadboard
Jumper wires
Getting Started
Wiring the Sensor: Connect the DHT11 sensor to your Arduino. Typically, the data pin goes to a digital pin (e.g., pin 2), while power (5V) and ground (GND) connect accordingly.
Using Libraries: Install the DHT11 library in your Arduino IDE to simplify sensor readings.
Programming: Write a sketch to read temperature and humidity values and print them to the Serial Monitor. Here’s a code snippet:
include "DHT.h"
define DHTPIN 2
define DHTTYPE DHT11
DHT dht(DHTPIN, DHTTYPE);
void setup() {
Serial.begin(9600);
dht.begin();
}
void loop() {
float h = dht.readHumidity();
float t = dht.readTemperature();
Serial.print("Humidity: ");
Serial.print(h);
Serial.print(" %\t");
Serial.print("Temperature: ");
Serial.print(t);
Serial.println(" °C");
delay(2000); // Update every 2 seconds
}
Uploading and Testing: Upload the code and check the Serial Monitor. You should see real-time temperature and humidity readings!
Why It’s Great
This project is not only practical but also helps you understand how to work with sensors. For example, diligent monitoring can reveal that indoor humidity levels should ideally be between 30% and 50% for comfort and health.
3. Ultrasonic Distance Sensor
Project Overview
Next, we will create a project using an ultrasonic distance sensor. This project is engaging because it measures distances and can detect obstacles, making it ideal for robotics enthusiasts.
What You Need
Arduino board
HC-SR04 ultrasonic distance sensor
Breadboard
Jumper wires
Getting Started
Wiring the Sensor: Connect the HC-SR04 sensor to the Arduino. The trigger pin connects to a digital pin (pin 9), and the echo pin connects to another digital pin (pin 10), along with VCC to 5V and GND to GND.
Programming: Write a program that triggers a pulse and listens for the echo to calculate distance. Here’s some basic code:
define trigPin 9
define echoPin 10
void setup() {
Serial.begin(9600);
pinMode(trigPin, OUTPUT);
pinMode(trigPin, OUTPUT);
pinMode(echoPin, INPUT);
}
void loop() {
digitalWrite(trigPin, LOW);
delayMicroseconds(2);
digitalWrite(trigPin, HIGH);
delayMicroseconds(10);
digitalWrite(trigPin, LOW);
long duration = pulseIn(echoPin, HIGH);
long distance = (duration * 0.034) / 2; // Calculate distance
Serial.print("Distance: ");
Serial.print(distance);
Serial.println(" cm");
delay(1000); // Update every second
}
pinMode(echoPin, INPUT);
}
Testing: Upload the code and open the Serial Monitor. Move your hand or an object close to the sensor, and you should see distance readings!
Why It’s Great
This project introduces you to ultrasonic sensors. According to studies, using distance sensors can enhance accuracy in obstacle detection in robotics by up to 90%. This is crucial for DIY robotics, where precise navigation is key.
4. RGB LED Color Mixer
Project Overview
A creative and colorful project is creating an RGB LED color mixer. This allows you to mix different colors using analog inputs, making it visually appealing.
What You Need
Arduino board
RGB LED
220-ohm resistors (for each color)
Potentiometers (for adjusting color intensity)
Breadboard
Jumper wires
Getting Started
Wiring the RGB LED: Connect the R, G, and B pins of the RGB LED to three PWM-capable pins on the Arduino (like 9, 10, and 11). Connect the respective resistors to ground.
Potentiometers: Connect three potentiometers to the analog pins (A0, A1, A2) to adjust color intensity.
Programming: Write a program that reads values from the potentiometers to control the RGB LED. Here's a helpful code snippet:
int redPin = 9;
int greenPin = 10;
int greenPin = 10;
int bluePin = 11;
void setup() {
pinMode(redPin, OUTPUT);
pinMode(greenPin, OUTPUT);
pinMode(bluePin, OUTPUT);
}
void loop() {
int redValue = analogRead(A0) / 4; // Scale to 0-255
int greenValue = analogRead(A1) / 4;
int blueValue = analogRead(A2) / 4;
analogWrite(redPin, redValue);
analogWrite(greenPin, greenValue);
analogWrite(bluePin, blueValue);
}
Testing and Mixing: Upload the code and turn the potentiometers to mix colors! Watch the RGB LED change as you adjust the values.
Why It’s Great
This project teaches the concepts of working with analog inputs and color mixing. Learning about color theories, like how mixing red, green, and blue in different ways produces various colors, is both fun and educational!
5. Simple Light Show
Project Overview
Finally, let's complete our journey with a simple light show project. This project combines everything you’ve learned to create a dazzling LED display.
What You Need
Arduino board
Multiple LEDs (different colors)
Resistors
Jumper wires
Breadboard
Getting Started
Wiring the LEDs: Connect several LEDs to different digital pins on the Arduino, ensuring each LED has a proper resistor.
Programming the Light Show: Write a program that lights up the LEDs in various patterns. Here’s a simple example:
int led1 = 2;
int led2 = 3;
int led3 = 4;
void setup() {
pinMode(led1, OUTPUT);
pinMode(led2, OUTPUT);
pinMode(led3, OUTPUT);
}
void loop() {
digitalWrite(led1, HIGH);
delay(500); // Stay ON for 0.5 seconds
digitalWrite(led1, LOW);
digitalWrite(led2, HIGH);
delay(500);
digitalWrite(led2, LOW);
digitalWrite(led3, HIGH);
delay(500);
digitalWrite(led3, LOW);
}
Testing the Show: Upload the code and marvel at the LEDs lighting up in sequence!
Why It’s Great
This project lets your creativity shine as you can create your own light patterns. Engaging in such projects reinforces what you’ve learned, making it an enjoyable way to apply programming skills.
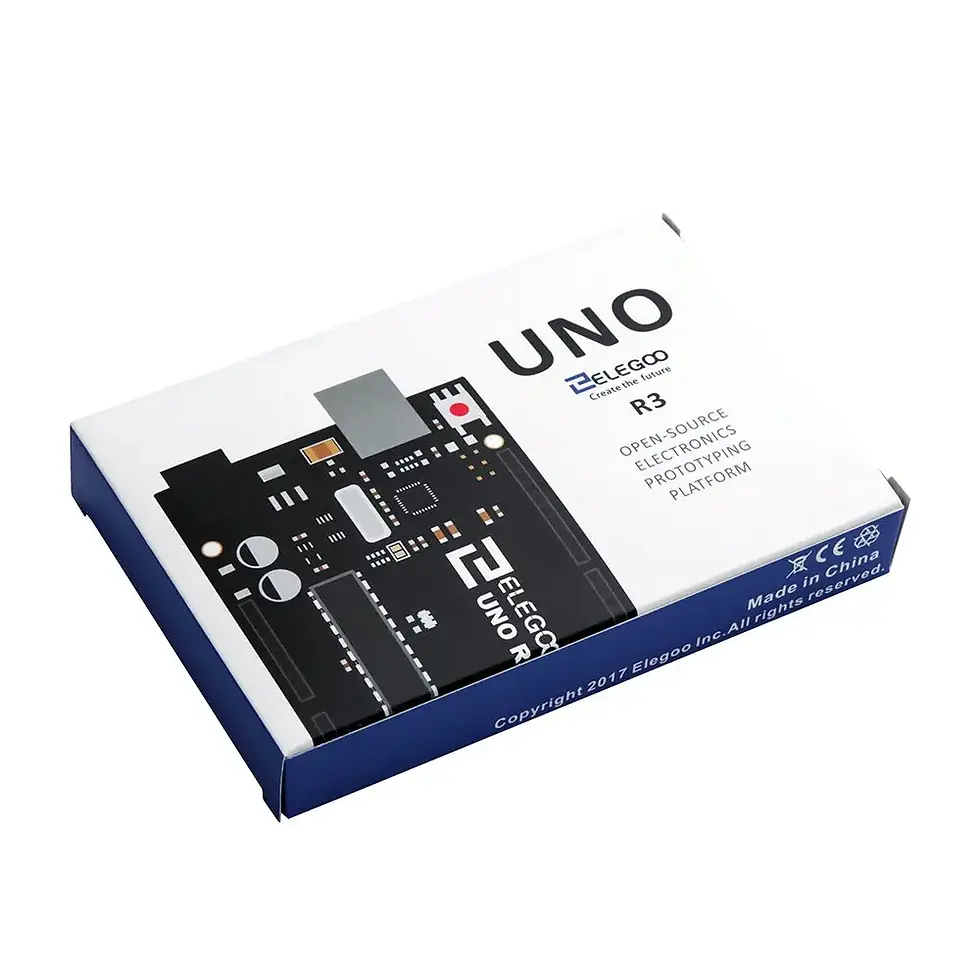
There you go! Five simple Arduino projects to kickstart your journey into the fascinating world of electronics and programming. Whether you want to light up an LED or create a full-fledged light show, these projects are valuable building blocks for more advanced work.
Arduino thrives on creativity, so feel free to modify these projects or innovate based on your interests. Happy tinkering!
Comments